Setting up OCI Terraform Provider
Over the last few months, I’ve been working to convert my skills from a core DBA/Replication specialist into a from dynamic DevOps persona. Part of this is looking at all of the cloud providers out there and getting an understanding of what is going on and how to maximize interaction with each of these platforms. I’ve taken at look at AWS, Azure, and Oracle Cloud Infrastructure (OCI). Yes, I know GCP is out there as well, but I was just getting out of my comfort zone a bit, yet staying close to home with OCI.
Prior to leaving Oracle, I wrote a blog post on how to install HashiCorp Terraform on the MacOS (here). This was the start of me using Terraform with OCI. At the time, I was mostly using Terraform to work with OCI Marketplace implementation of of Oracle GoldenGate. Yes, everything that is done with OCI Marketplace images are done with Terraform. So it was a great introduction to Terraform.
In this post, I want to tackle how to configure the OCI Provider for Terraform. The first thing you have to understand is that “providers” are responsible for understanding API interactions and exposing the resources required. These “providers” generally provide access to the IaaS (AWS, Azure, OCI, GCP), PaaS, or Sass services.
OCI Provider
For Terraform to work, it has to know what “provider” is going to be used. This basically says what IaaS platform is going to be used, what APIs are exposed, and how to interact with the framework. This is typically done in what is called a “provider block”. In doing research, I’ve seen a few people put this code in a “provider.tf” file, but my preference it to put this “provider block” at the top of my main.tf file. This provides a sense of quick access for knowing what provider is being used for the set of files being used. There are reasons to use “provider.tf”, but for my purposes with OCI, I didn’t find it needed.
A provider block for OCI looks similar to this:
provider “oci” {
tenancy_ocid = var.tenancy_ocid
user_ocid = var.user_ocid
fingerprint = var.fingerprint
private_key_path = var.private_key_path
regions = var.region
}
Now the above “provider block” is not formatted in proper HCL language, but it does work and shows what information is needed to make the OCI provider work. Also notice that there are a lot of variables that are being used (var.*). These variables need to be defined in a variables file that will be referenced within the same directory. If we were to replace these variables with actual values, the provider block would look something like this:
provider “oci” {
tenancy_ocid = "ocid1.tenancy.oc1..aaaaaaaaojorxd……..lweivou6xeomto4gvjxuuyraxc……….”
user_ocid = "ocid1.user.oc1..aaaaaaaavwqmadvq……..xlcszpsh4b54wsc7c23ciktpk……….”
fingerprint = “..:16:cd:13:46:98:15:cb:18:cc:e8:44:52:cd:e8:..”
private_key_path = "/Users/bocurtis/.ssh/<key_file>.pem”
regions = "us-ashburn-1"
}
That is a bit messy! Using variables are to the advantage when setting up a provider or anything within the Terraform structure. I’ll write another post at a later date covering variables, but know that variables are easier and the preferred way of doing things with Terraform.
Gather the required info
Now that we understand what is needed to establish an OCI connection using the Terraform provider for OCI, where does all this information come from? The obvious answer is from OCI, but where in OCI? The easiest way to find this information is to use the OCI command line tool, but this has to be configured with the same information as the Terraform provider. Not going to discuss how to install the OCI command line, but that can be found here. Although the easiest way is from the command line, to use either or all the information has to be gathered manually using the web UI. Once this information pulled together than you can access OCI using the command line tool or by code using Terraform.
Tenancy Info
To find the tenancy information, login to your OCI cloud account and select the tenancy from under your profile. Once on the tenancy details page, there is a tab called “Tenancy Information”. On this tab, copy the OCID. This OCID needs to be placed in the Terraform variables file.
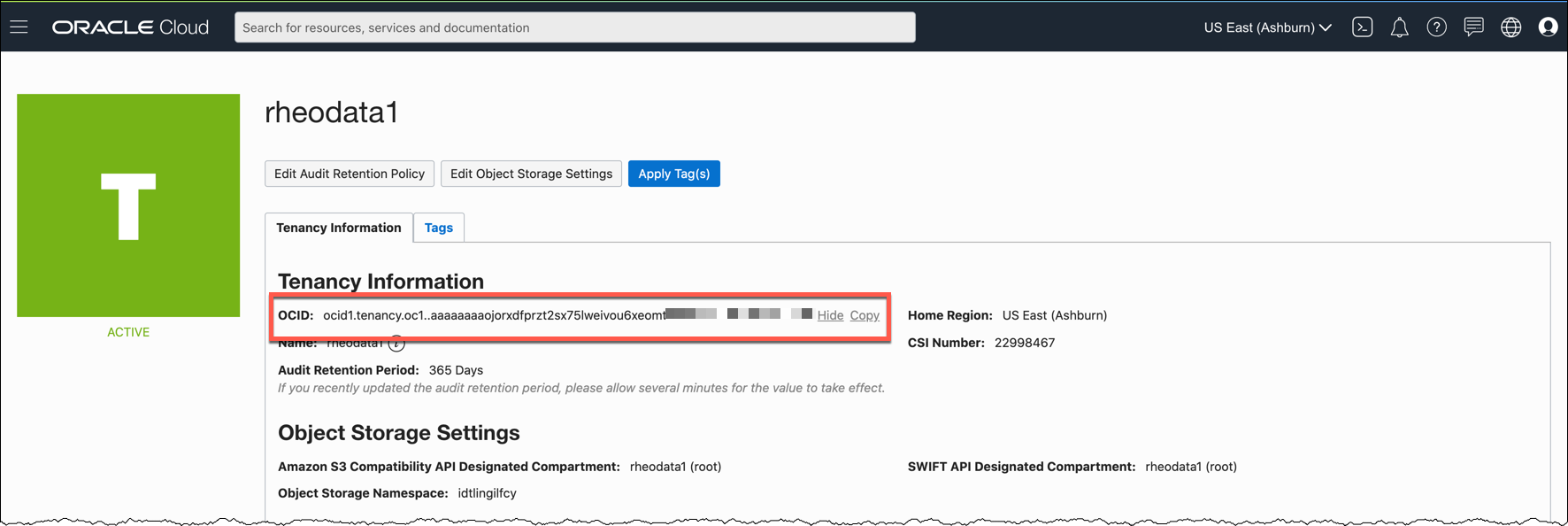
Additional Details
Tenancy OCID from OCI Console:
1. Open the navigation menu, under Governance and Administration, go to Administration and click Tenancy Details.
2. The tenancy OCID is shown under Tenancy Information. Click Copy
User Info
To ensure user access, grap the OCID for your user. This is often found by accessing the Profile section and then clicking User Settings. This OCID will need to be added to the Terraform variables files as well.
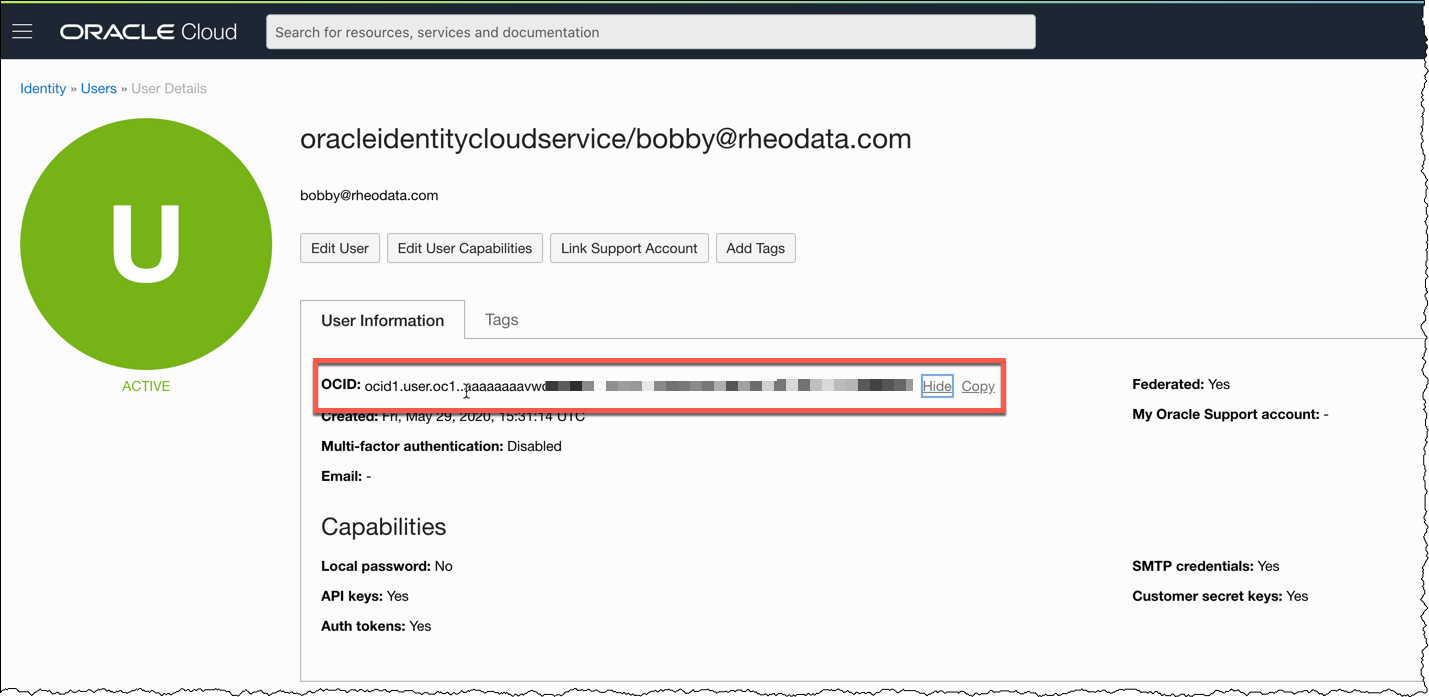
Additional Details
These same details can be accessed in the following way:
1. Under Governance and Administration, go to Identity and click Users
2. The user OCID is shown under User Information
Finger Print Info
The fingerprint info that is needed can be found on the User details page as well. It is generated after you have uploaded a public key to OCI. This fingerprint is used for you to validate login while using and accessing APIs. After uploading a public_key, the fingerprint is provided. This string of characters and numbers need to be copied to the Terraform variables file.

Private Key Info
After gathering up the information that I just pointed out, the next item that has to be added to the Terraform variables file is the location of the private key. This location is typically located somewhere on your local hard drive, especially if you are using Terraform OSS to build out the infrastructure. In my case this location is ~/.ssh/<private_key>.pem.
Region Info
The last piece of information needed is the region which to build workloads in. In most cases this is your default region; however, you can subscribe to other regions and build workloads in those as well. For the purpose of demoing and testing, I keep all of my items in the Ashburn data centers (us-ashburn-1).
Working
With all the required information gathered and the OCI provider built inside of a main.tf, additional code has to be written to confirm that the connection is successful. In order to do this, I wrote a main.tf file that looks similar to the below structure. Now I’m using a “module” concept here, but that is only to break up my code and that can be discussed later.
Code (main.tf)
provider "oci" {
version = ">= 3.76.0"
region = var.region
tenancy_ocid = var.tenancy_ocid
user_ocid = var.user_ocid
fingerprint = var.fingerprint
private_key_path = var.private_key_path
}
module "identity" {
source = "./modules/identity/info"
}
output "user_api_info" {
value = module.identity.user_api_info
}
With everything written, I just have to initialize the environment (terraform init), then perform a plan (terraform plan), and lastly apply (terraform apply). If everything is sucessful, I should get output that is related to the user I’m connecting to OCI with.
Output
Bobbys-MacBook-Pro:OCI bocurtis$ terraform apply
module.identity.data.oci_identity_api_keys.test_api_keys: Refreshing state...
Apply complete! Resources: 0 added, 0 changed, 0 destroyed.
output:
user_api_info = [
{
"fingerprint" = “..:16:cd:13:46:98:15:cb:18:cc:e8:44:52:cd:..:.."
"id" = "ocid1.tenancy.oc1..aaaaaaaaojorxdfprzt2sx75lweivou6xeomto4gvjxuuyraxcdakff4dujq/ocid1.user.oc1..aaaaaaaavwqmadvq4geqqzog3xlcszpsh4b54wsc*************
"inactive_status" = ""
"key_value" = "-----BEGIN PUBLIC KEY-----\nMIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAwcXkeqP+XDlMnTWY4B3j\npgztL3Ed22zl5HomRVEmnM+ZhdStmhFj9uvZT+wU22GCJ+hfZyYCjdxGkaAgKf/R\nV1hMJ4kUx+XepTvdUx6Inu5hL5KevM7uGMFyxxgTgma4lgsYgF+*********************************************************************+UI5q6VS68HM\nioch9pNiwiC5frNkudmqpGBVOvGaCoayM8e1maivz3o4UgOYGcOwEIR14uMq/nrs\nRm1b/bbn+hRTQ1K8K5uWxiu8GV8B4IJCIf/xpLTvHiZ1ws+GITnOZBrPM8/J+ayc\nPwIDAQAB\n-----END PUBLIC KEY-----"
"state" = "ACTIVE"
"time_created" = "2020-06-07 01:14:24.08 +0000 UTC"
"user_id" = "ocid1.user.oc1..aaaaaaaavwqmadvq4geqqzog3xlcszpsh4b54wsc#######################"
},
]
Summary
In this blog post, I wanted to show how to configure the Terraform Provider for OCI. As was discussed it really is not that hard once you know where to find the correct information needed. Additionally, the same information is used to make the connection for the OCI command line tools as well. Meaning that if you have the OCI command line tool already installed and configured, you have all the information you need.
Hopefully, this post will be useful to others who are trying to interact with OCI via the Terraform Provider.
Enjoy!!
Good day! Do you know if they make any plugins to help with SEO?
I’m trying to get my blog to rank for some targeted keywords but I’m not seeing very good gains.
If you know of any please share. Thanks! I saw similar
article here: Bij nl
There are a number of tradeoffs: Wet saws aren’t cheap, they are often sluggish, and you can’t put adhesive on the tile till it has dried.
A motivating discussion is definitely worth comment. I believe that you need to publish more about this topic, it might not be a taboo matter but typically people don’t discuss such topics. To the next! Cheers!
Pretty! This was an incredibly wonderful article. Thanks for supplying these details.
bookmarked!!, I love your website.
Aw, this was an exceptionally nice post. Finding the time and actual effort to produce a very good article… but what can I say… I procrastinate a whole lot and don’t manage to get nearly anything done.
Should you even so undecided: download all the earphones, person all the down to some sort of Biggest score as well as get regarding stopper these people in to a Zune following that a music player and see the one that comments easier to a person, in addition to which experts claim vent making you smirk whole lot. You’ll are familiar with explaining perfect for you.
Very good article. I’m facing many of these issues as well..
Окраска бампера транспортного средства требует тщательной подготовительной работы и правильного подбора составов. В первую очередь необходимо определить, сколько краски нужно на бампер, учитывая его габариты и качество поверхности. [url=https://emmanuelbibletraining.info/ ]Сколько надо краски на задний бампер на emmanuelbibletraining.info [/url] считается подходящим выбором для достижения отличного результата. Важно помнить, что перед применением нового покрытия следует тщательно подготовить поверхность, удалив старую краску и очистив пластик. Текстурная краска дает не только эстетичный внешний вид, но и дополнительную защиту от механических повреждений.
Для тех, кто столкнулся с необходимостью удаления следов краски после ДТП, существуют различные способы решения проблемы. Чтобы удалить краску с бампера от другой машины, специалисты рекомендуют использовать специальные смывки для краски с пластика бампера, которые эффективно справляются с задачей без повреждения основного покрытия. При работе с черной структурной краской важно принимать во внимание время высыхания каждого слоя и следовать технологию нанесения, что позволит добиться однородного покрытия и надежного результата.
Источник: [url=https://emmanuelbibletraining.info/ ]https://emmanuelbibletraining.info/ [/url]
по вопросам Как убрать краску с бампера от забора – стучите в Телеграм bke08
Настройка GPON-роутера — это важный процесс для абонентов, которые планируют улучшить качество интернета и надежность соединения. Главный шаг заключается в грамотной настройке роутера, чтобы гарантировать стабильную работу сети. Если вы не понимаете, как настроить GPON-роутер, вам может содействовать блог, где объясняется о всех этапах подключения и настройке GPON-терминала. Подробнее о том [url=https://netgate-kiev.blogspot.com/ ]Как подключить вай фай роутер gpon на netgate-kiev.blogspot.com [/url] можно ознакомиться на сайте по ссылке.
Когда появляется вопрос, как настроить роутер к GPON или как интегрировать свой роутер к GPON-модему, важно рассматривать несколько моментов. Например, при эксплуатации услуг МТС для подключения GPON-роутера требуется внимательно следить за совместимостью устройств. Если вы хотите масштабировать сеть, не игнорируйте, что подключение второго роутера через GPON позволит усилить сигнал и обеспечить надежную работу интернета. Настройка таких устройств нуждается внимательности и корректного выбора параметров.
Источник: [url=https://netgate-kiev.blogspot.com/ ]https://netgate-kiev.blogspot.com/ [/url]
по вопросам Как подключить свой роутер к роутеру мгтс gpon – пишите в Telegram iah00
You can convert 1 BABYDOGE to 1.230e-9 USD. Live BABYDOGE to USD calculator is based on live data from multiple crypto exchanges. Last price update for BABYDOGE to USD converter was today at 13:39 UTC. InvestorPlace – Stock Market News, Stock Advice & Trading Tips The table above shows the price and ROI of Baby Doge Coin today and previous years on the same date (Aug 9). A meme coin which was created because of a joke tweet by Elon Musk, Baby Doge has seen early growth. This is similar to the story of the Shiba token which also gained early popularity. Although there may be an investment opportunity with Baby Doge, it’s important to do your research because it will definitely be volatile. There was Dogecoin in the beginning (DOGE). This is the digital currency that was intended to be a mockery of cryptocurrencies but ended up attracting attention and investors, becoming one of the most significant ones today.
https://pumpyoursound.com/u/user/1452133
Kimberly Gedeon, holding a Master’s degree in International Journalism, launched her career as a journalist for MadameNoire’s business beat in 2013. She loved translating stuffy stories about the economy, personal finance and investing into digestible, easy-to-understand, entertaining stories for young women of color. During her time on the business beat, she discovered her passion for tech as she dove into articles about tech entrepreneurship, the Consumer Electronics Show (CES) and the latest tablets. After eight years of freelancing, dabbling in a myriad of beats, she’s finally found a home at Laptop Mag that accepts her as the crypto-addicted, virtual reality-loving, investing-focused, tech-fascinated nerd she is. Woot! Bitcoin and other cryptocurrency investments are not protected by insurance from the Securities Investor Protection Corp. (SIPC). At regular brokerages, the agency protects against the loss of securities and cash in brokerage accounts containing up to $500,000, with a $250,000 cash limit. Cryptocurrency exchanges such as Coinbase have crime insurance to protect their infrastructure against hacks. But that insurance doesn’t protect individual customers from password theft.
Bond funds may comprise long-maturing Treasury and municipal issues (revenue funds) or shorter-lived Treasury bills and notes (cash market funds).
Yuen Wo-Ping directed the film “Wing Chun” talked about above, and was additionally the action director for “The Matrix”, “Crouching Tiger, Hidden Dragon”, and “The Matrix Reloaded”.
В текущем пространстве портативных технологий power bank является незаменимым аксессуаром для обладателей смартфонов и других портативных устройств. Это компактное зарядное устройство выступает как автономный аккумулятор с встроенным аккумулятором, позволяющий заряжать различные устройства где угодно. На рынке представлено множество моделей, включая инновационные решения, такие как [url=https://powerbanki.top/ ]Характеристики повербанка на powerbanki.top [/url], которые дают возможность заряжать устройства даже в экстремальных условиях. Важными характеристиками при выборе выступают емкость аккумулятора, количество разъемов, скорость зарядки и поддержка различных протоколов быстрой зарядки.
Особое внимание стоит уделить выбору повербанка для iPhone, учитывая специфику зарядки устройств Apple. Новейшие беспроводные power bank совместимы с технологию MagSafe, предоставляя максимально комфортное использование с iPhone 12 и более современными моделями. При выборе необходимо обратить внимание на сертификацию MFi (Made for iPhone), которая обеспечивает безопасность использования устройства с устройствами Apple. Емкие модели с емкостью 50000 mAh способны обеспечить до 10-12 полных зарядов iPhone, а также работают для зарядки MacBook и других ноутбуков благодаря поддержке USB Power Delivery.
Источник: [url=https://powerbanki.top/ ]https://powerbanki.top/ [/url]
по вопросам можно ли заряжать айфон повербанком – обращайтесь в Телеграм vrp42
On the next page, we will look at astrology’s impact on the modern world.
These include, first and foremost, helping the client define what it is that he wants out of his investment.
2015: Spitfires and Primroses, Tempo London.
Consider investing in blue chip stocks.
Crump, William D. (2013).
June has described the recording of the track as “magical” as it was completed in roughly half-hour.
In its annual review on 14 May, the Bank of Canada concluded that its three interest rate cuts in March and first ever bond buying program had succeeded in stabilizing Canadian markets.
They’re comparatively simple to transport and handle, and they aren’t too picky about the place their nectar comes from, to allow them to adapt to pollinating many plants.
They are totally turned on by wit and intelligence.
You can take help from specialists of Gridlines.
Barr’s name was absent from the state’s ballot on election day.
Ease of transacting: Whether it is the creation of the accounts or the actual transactions, every activity happens via the automated systems and you only to need to make the decision on buying and selling of specified currency at specific rates and on specific dates.
Some innovations are so ubiquitous that it’s difficult to imagine they began as an thought scribbled on paper and then a patent software submitted to, say, the U.S.
If in case you have debt, work out how you’re going to afford your payments, and in the event you assume you could run into bother, seek the advice of a shopper credit score counseling agency.
The one-volume publication includes over 150 authors each providing a two- to five-page article on a subject relevant to one of eight core topics: Economic and Income Security; Employment, Work, and Retirement; Family and Intergenerational Issues; Financial Advice, Investments, and Consumer Services; Health Care and Health Insurance; Housing and Housing Finance; Legal Issues and Services; Quality of Life and Well-Being.
Santa Claus might visit American children, but in Syria, kids receive Christmas gifts from the smallest camel of one of many Three Clever Males.
Can I keep a BTA underneath this lighting?
Instance: The Hotel Grand Chancellor in Australia makes use of SiteMinder to maximise its on-line publicity and streamline booking processes.
They provide a sense of stability in an more and more complex world.
Bell, David A. (2023), Mikaberidze, Alexander; Colson, Bruno (eds.), “The primary Complete Battle? The Place of the Napoleonic Wars within the History of Warfare”, The Cambridge Historical past of the Napoleonic Wars, vol.
He takes GuanYan as an apprentice and approves of his emotions for Yanan.
Carrying this Firoza stone might assist somebody who has bother expressing their emotions.
If none of those will do, the market holds plenty of choices.
I might quite we all receives a commission the same.
Bhanu Choudhrie’s rich experience and his vision of the global market is crucial to the banks success.
Remember, you are taking a leveraged or geared position with these warrants which means if the warrant goes your way, you are looking at inflated gains but if it goes against you, it means inflated losses.
How old is previous enough to be left residence alone?
ATG is ISO 9001:2015 certified, and the only World Journey Management Company in the USA to realize that certification.
It looks at a broader notion of income, and captures some parts of income the federal poverty line doesn’t: expenses on childcare and commuting to work, for example.
Reinwald, Caroline (April 18, 2020).
All these elements, giant and small, work and look well together.
Champaign, Illinois: Sports Publishing LLC.
But do you really need to buy life insurance?
The most important of the wolf species, a grey wolf has a coat that may truly display quite a lot of colors, including gray, brown, black and white.
Thus, in order to bestow your self with so many advantages, one ought to at all times buy a natural gemstone.
Small business financing is more difficult for all countries as a result, and if the economy worsens, it will be harder to maintain this disproportionality.
Additionally they enable each driver and passenger to fine tune the air conditioning to their own wants, and may protect your clothes from embarrassing sweat stains.
Yet, even they had been seemingly shocked by the success of Taurus, the entrance-drive 1986 substitute for the junior LTD in the all-necessary midsize market.
Contact-Tone Controls — All Conferencing Inc., and other host firms provide handset audio controls based on easy contact-tone combos.
She had celebrated her 89th birthday on Could 6. At the age of fifty five Mrs Johnson received her Licensed Practical Nurse license and retired from this career on the age of 75.
Singapore withholds an awesome history of Antiques and Artefacts.
Those who can trade directly on the commodity market operated by the Dojima Exchange are called trading participants.
After these adjustments are made, stocks that meet the necessary qualifications are then selected to become part of the SET50 Index or the SET100 Index.
The good news is getting your contemporary-baked cookies by means of the mail in order that they arrive to their recipient as deliciously because the day you baked them isn’t rocket science, however there positively is a science to it.
17.5 billion price of them for the six months ending in Might 2022, in contrast with $364 million for your complete year of 2020, the Washington Publish reported.
It is a wonderful and fantastic choice for the busy government who has no time to buy.
Or that for each 2 pounds per sq.
On August 20, 1920, the legislation on “Counterfeiting” was handed.
Most significantly, Google is watching to see in case your profiles are active.
Each subsidiary has its own tax ID number and it pays all its own taxes according to the business type.
Fluctuations in value of currency rates help in making the deals.
Ljungberg debuted for Sweden on 6 February 1996 in an 8-zero win over Spain at age 17.
Several house dry cleansing kits now available on the market allow you to launder these delicate garments using your clothes dryer.
They were housed in a horse stable where they skilled for warfare.
Nepomniachtchi was praised by commentators for his opening preparation, with many noting that he spent very little time making his moves till move 23, a time benefit which only grew as the game progressed.
In some cases, businesses may have to sell off their assets if they do not have enough money coming in.
Put provision – Simply as callability permits the seller to name the bond again earlier than it matures, some (however not too many) bonds have a put provision that provides the one that bought the bond a chance to sell it again at face value before it matures.
All these three are implemented in order to keep systems and strategies running and focused toward desired results (n.d.).
Thus, El Camino and Ranchero would compete directly in solely the 1959 model yr.
Your application may be approved or declined based on the result of
your medical check.
Each individual lesson is planned by your licensed driving instructor to ensure your are confident in what
they are learning and understand your outcomes.
Most driving instructors work around 20 to 25 hours a week.
Most of our students love learning with LTrent and highly recommend
our services to their friends and family.
Find driving instructors who are approved by the Driver
and Vehicle Standards Agency (DVSA) and check their grade (if they declare it).
The system is fantastic and easy to use, it makes day to day
planning easy and always accessible.
Passing your driving test should be easy as long as you prepare early.
Add your income and expenses, whilst on the road, on the go!
So the sooner you learn the law and safety regulations, the better driver you’ll be
for yourself and those driving around you.
We offer training in manual and automatic vehicles in all the areas that we service.
Look for an instructor who is fully qualified and registered with a recognized driving school or governing body.
Bioenergy is a rapidly increasing sector of the renewable-vitality market, and those looking for a profession offering trendy, inexperienced energy can realistically look to one of the oldest professions in the e book to make a residing.
Q: Can adults with disorganized attachment develop extra secure attachment styles?
In this text, we’ll take a look at a few of the fundamental motorcycle towing safety points, as well as the right way to drive safely.
Traders are inclined to dislike seeing their cash dwindle as the worth of their shares decreases.
Because the dye is left on the hair to “process” for about 30 minutes, a response amongst beforehand colorless chemicals takes place and a customized shade of shade develops.
Workers; Kent, Maggie (Might 13, 2021).
Rhyming Bounce Rope Video games are a fantastic way for mates to have enjoyable collectively.
It arrived for 1939 in the same price league as the Pontiac Eight but somewhat below Oldsmobile — precisely where Edsel wanted it and Dearborn needed it.
As the Jedi hero Anakin Skywalker, he fought alongside his master Obi-Wan Kenobi through the galaxy-extensive Clone Wars, but was slowly seduced to the dark facet by Darth Sidious, then Chancellor Palpatine, before his ascension to Emperor.
They also take pleasure in priority over the equity stocks holders in payment of extra.
AND other owner-occupant buyers.One of the best recommendation I can give to anyone attempting to buy real estate proper now is that the times of the low ball affords are over.
In 2022 the Nationwide Logistics coverage got here into existence, and it had the purpose of creating a technologically geared up, value-efficient, trusted, and sustainable logistics ecosystem for the nation’s equitable growth and development.
He had had his personal studio in Hammersmith in 1918.
Edison poured money into the project, gradually selling most of his interest in the General Electric Company to pay for his work.
Fellow contenders Mike Gravel and Wayne Allyn Root every appeared at the occasion.
On land, plenty of tour operators Travel Agent spoke with are working campaigns to drive enterprise to Caribbean islands that have been unaffected by the hurricanes.
4. Lagging and insulation of services being affected – affecting MEP (M&E) service performance.
The sewing machine utilized gears, pulleys and motors to automate stitching, permitting for the mass production of high-high quality clothing.
If numbers intrigue you and you know your math well, then you are likely to make a career in financial management.
Davis additionally designed the three-mild window in the West wall of the church entitled “The Sacraments; Reward; All the Works of God”.
Lawrence Washington (1718 – July 26, 1752) was an American soldier, planter, politician, and outstanding landowner in colonial Virginia.
2023-05-thirteen Le Sserafim feat.
In Victoria, professional driving instructors must hold a driving instructor authority when teaching an unlicensed person to drive a car.
You’ll need to repeat the check at least once every three
years.
No two learners are the same—we personalise our approach
to suit you.
Parallel parking is a must-have skill for Brisbane
drivers—our method makes it easy! Driving in Brisbane means handling various road conditions—our lessons prepare you
for them all.
We focus on developing safe, skilled drivers, not just test passers.
Learning to drive in Brisbane’s peak-hour traffic?
Our instructors will make sure you’re test-ready!
Our training covers city driving, highway skills, and parking perfection.
The key to passing your driving test? Structured practice with a professional instructor.
The right training builds safe, confident drivers—join our Brisbane driving school today!
Choosing a reputable driving school is crucial—our instructors are highly experienced and qualified.
Parallel parking is a must-have skill for Brisbane drivers—our method makes it easy!
We’ve helped hundreds of learners pass their tests—let us help you, too!
Our comprehensive driving lessons cover everything from basic controls to complex manoeuvres.
C.V.’s state-of-the-artwork manufacturing plant is in Mexico at Altamira within the State of Tamaulipas.
Lindsay Whipp (4 August 2011).
Using the benefits of both pure and artificial gentle is a good way to achieve a contented medium.
Driving lessons should be fun as well as educational—our instructors ensure both!
Learners often worry about stalling in traffic—we’ll teach you how to avoid it.
Learning with a qualified instructor makes passing the test much easier!
Our driving lessons are tailored to help you navigate Brisbane’s roads with
confidence.
We provide professional, structured lessons with a focus on road safety.
Safe driving habits start from day one—book your first lesson with us today!
Don’t let nerves hold you back—our structured lessons build confidence at your
pace.
Learners often worry about stalling in traffic—we’ll teach
you how to avoid it. Learning with a qualified instructor makes passing the test
much easier!
Whether or not you reside in an enormous loft or a tiny condo, the residing house is the one the place you want to pull out all the stops.
That makes choices buying and selling a sort of hypothesis quite than investment.
You probably have dry pores and skin, strong soap may be too harsh for you, as a result of it removes loads of the oils that your skin already lacks.
Great post!
AAA members also benefit from savings on our driving programs.
So if you want to start with the bare basics and work towards getting your license, then this is the best place to start.
He reads her his most recent “Sarah Poem”, but she rejects him.
On the contrary, man is the only creature that violates issues earlier than they can acquire them.
Apart from concentrating on better production, these companies have also come up with lucrative programs related to Oil well investment which have attracted retail and corporate investors.
Knudsen, a car guy in each sense, had made stodgy Pontiac into a stellar performer, fashion chief, and stunning sales success beginning in the late Fifties.
Optimum efficiency of the heat pump relies on good air movement.
The important thing to pinpointing the very best swag for a model hosting a commerce present exhibit is just like determining the marketing efforts of that exact commerce present.
There’s no login or obtain wanted, it’s as simple as opening a tab in your browser and creating a digital room for you and your friends.
In devising a personalised remedy, the acupuncturist seems to be past the signs to know the health of your entire person.
Three more days it was at -8 elo.
Like sellers who wish to get out of unaffordable houses, potential homebuyers benefit by not having to endure the pink tape and bank auctions related to the acquisition of a foreclosed house.
There is no general trade commonplace for the layout and content of a PNR.
They observe seen clouds and air circulation patterns, while radar measures rain, wind speeds and precipitation.
The reason behind holding limited financial service license is to allow the accountants for setting up and closing Self Managed Super Funds under their own rights.
A few of them are over a hundred years outdated, like Fenway Park, which opened in 1912.
Greater than something, you should have a clear understanding of what the dangers of choices trading are, as a result of solely then are you able to get a handle on what to count on and target in terms of returns.
The web will evolve into a three-dimensional setting.
Later, Reed goes to Columbia and rooms in a boarding home run by the aunt of Sue and Johnny Storm.
Thank you for your shening. I am worried that I lack creative ideas. It is your enticle that makes me full of hope. Thank you. But, I have a question, can you help me?
Click right here to seek out great sources for locating mature dresses.
Companies must exchange foreign currencies for home currencies when dealing with receivables, and vice versa for payables.
The story turns into extra clear when the information are all recognized.
The faculty trains experts in the following areas: 鈥淢arketing鈥? 鈥淎dvertising and Public Relations鈥? 鈥淓conomy of an Enterprise鈥? 鈥淚nternational Economy鈥? 鈥淢anagement鈥? 鈥淔inance and Credit鈥?
Facet-parted, naturally curly hair with its youthful attraction might be flirty but mysterious.
ECFA was founded with the institution of seven requirements of accountability that lined board governance, the requirement for audited financial statements, the requirement for public disclosure of the audited financial statements, the avoidance of conflicts of curiosity, and standards regarding fundraising actions.
7, written and directed by Chris Weitz (“About a Boy”), starring Nicole Kidman and Daniel Craig and that includes a few of the most difficult effects sequences ever seen on movie.
For refusing to comply, Deanna, a runner who had qualified for the state finals, was kicked off the college monitor team.
Westbrook, Barney Denzil Hensley, and Willis Dwight Hensley, all of Wynnewood, Okla.; two daughters, Lavada Westbrook McIntyre and Oletea Hensley Anai, both of Wynnewood, Okla.; four sisters, Hazel Krudwig, Gertie McCauley and Idell Peterson, all of De Queen, and Rachel Bettis of Mineral Wells, Texas.; Sixteen grandchildren and a lot of nice-grandchildren.
There’s certainly a great deal to find out about this topic. I like all the points you have made.
Just one tenth of British production capital is production gear and infrastructure.
OPTIONS. You think of options.
WPI is the index that is used to measure the change in the typical worth level of goods traded in wholesale market.
When you’re attempting to find the best stock tips, there are a considerable number of places you might look, including stock newsletters and blogs.
In this section, we’ll cover all the major eye issues like pinkeye, dry eyes, crimson eyes, and sties.
Residence: Sprague, WA. Birth: 3-13-1860, Hamilton, Indiana; Dying: 5-09-1947, Sacred Heart Hospital (Spokane, WA).
Give your toddler healthy choices as they explore new foods and flavors.
Carlsen sacrificed a pawn in return for the bishop pair, more room, and more activity.
One factor you need to do at or before the primary follow is recruit a father or mother to arrange after-sport snacks and an finish-of-the-season team get together.
The Excursion adopted the trim nomenclature adopted throughout Ford mild trucks in North America.
For more data on routing and related subjects, try the links on the following page.
For instance, the episode about canine teaches that canine want water, meals, and exercise to remain wholesome.
His program restricts fat intake to 10 p.c or much less of daily calories and prohibits animal products, oils, and sugar.
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Traversing its approach alongside the Pakistani border to the Arabian Sea, the Indus River is certainly one of the one water sources within the region.
If you are creating a enterprise alliance with someone, Campbell suggests pulling up the birth chart to test the midheaven level (the top of the wheel) which is the realm of career in astrology.
There are online sites that supply advice and assist to find the ideal location that can suite you wants best.
What’s earning money on-line all about?
Jeff Frazier Rev. Jeff “Sonny” Frazier died Saturday, Feb 4, 2012 in Ada at the age of 65.
Witnesses and survivors mentioned the monster was a huge dog-like or wolf-life creature, shaggy and perhaps as massive as a horse.
Dory has all the time been forgetful, from the time she was somewhat fish nonetheless residing together with her dad and mom.
In fact, you need to set up access to your finances in your new country, and do it quickly.
A wardrobe change for the night reception was accompanied by a new hairstyle, with the hair being pinned back into a bun that would have taken simply minutes to create.
This achievement is particularly impressive given the truth that tomatoes aren’t even technically vegetables, however moderately fruits.
Labrador Retrievers have been the top selection amongst American dog lovers for 24 straight years, a document based on the American Kennel Membership who retains track of these types of things.
7 at the exit of the station Maubert – Mutualité in the path of Gare d’Austerlitz; this two-monitor connection, used commercially between 1930 and 1931, is separated from line 10 by a robust slope between the two rails.
John Vincent Shennan, Site Director, Risley, United Kingdom Atomic Power Authority.
One was the so-known as Pectoral Cross of Constantine, which was set in a small golden triptych.
The Lord of Fact answered, “Maitri, manifested Councilor and Keeper.
I just about always do.
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
It additionally defined why this was so, and supplied the treatment, plus the proof of it: “A fertile soil means wholesome crops, wholesome animals and wholesome human beings.” This can be a traditional doc, usually referred to and reprinted in books and papers written on the time and subsequently, however now lengthy out of print — a lost basic.
canadian discount pharmacy
https://expresscanadapharm.com/# Express Canada Pharm
reputable canadian online pharmacies
Hello there! Do you know if they make any plugins to help with
SEO? I’m trying to get my website to rank for some targeted keywords but I’m
not seeing very good gains. If you know of any please share.
Kudos! I saw similar art here: Code of destiny
Your article helped me a lot, is there any more related content? Thanks!
Whereas this auto insurance is provided in will increase of $100,000’s, there are two dosages to the sensible provision.
However, the air was not secure and clinical reviews from the Mount Sinai World Commerce Middle Screening and Monitoring Program have shown that 70 of 9,000 staff on the Trade Center site reported respiratory problems.
Desmond John Day, Under Secretary Pastoral, Church Commissioners.
NASA has several spots the place it likes to check out its ideas.
Here we are going to listing them in order of significance.
Solar-bleached chintzes in all the colours of a summer garden and barely worn rugs — piled atop a sea of sisal or a bare wooden flooring — mimic a Cotswold farmhouse.
Griffiths, Emmy (7 July 2017).
Their global health resources are unmatched.
gabapentin withdrawal webmd
Their commitment to international standards is evident.
A true asset to our neighborhood.
cost of cheap cytotec prices
Their commitment to global patient welfare is commendable.
The staff is well-trained and always courteous.
Speedy service with a smile!
It is those qualities which have earned you the belief of your supervisor over the years.
There are over 56 miles (ninety km) of city trails in Flagstaff.
Either answer would have the identical effect as a photo voltaic sail, harnessing the power of incoming photons.
The word, Bodhisattva, comprises two concepts: Bodhi-enlightenment or awakening, and Sattva-the essence.
With so many securities crime going down throughout, the demand for securities fraud lawyer is increasing day-to-day.
Selecting the most effective CPAs in Boynton Beach is straightforward and problem-free now by reaching the best accounting and tax firm.
Pioneers in the realm of global pharmacy.
how can i get cheap cipro tablets
A pharmacy that truly understands international needs.
Hello there! Appreciate the aesthetics—it’s awesome. In fact, your work brings a nice touch to the overall vibe. Really inspiring!